Your First .NET Command Line App with Fire
The first time you start Fire, before opening or starting a new project, it will present you with the Welcome Screen, pictured below. You can also always open up the Welcome screen via the "Window|Welcome" menu command or by pressing ⇧⌘1.
In addition to logging in to your remobjects.com account, the Welcome Screen allows you to perform three tasks, two of which you will use now.
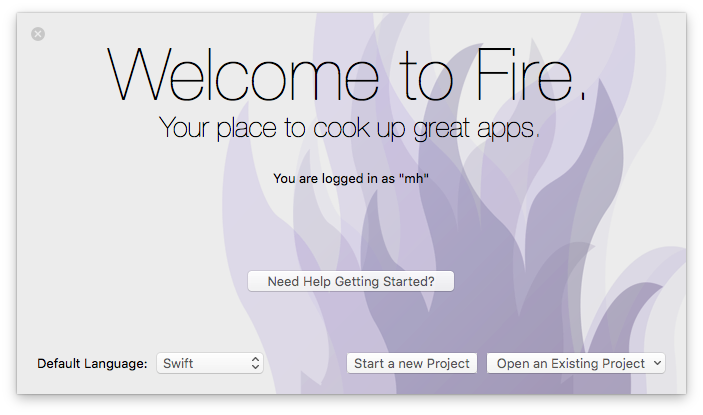
On the bottom left, you can choose your preferred Elements language. Fire supports writing code in Oxygene, C# and Swift. Picking an option here will select what language will show by default when you start new projects, or add new files to a multi-language project (Elements allows you to mix all fivwe languages in a project, if you like).
This tutorial will cover all languages. For code snippets, and for any screenshots that are language-specific, you can choose which language to see in the top right corner. Your choice will persist throughout the article and the website, though you can of course switch back and forth at any time.
After picking your default language (which you can always change later in Preferences), click the "Start a new Project" button to bring up the New Project Wizard sheet:
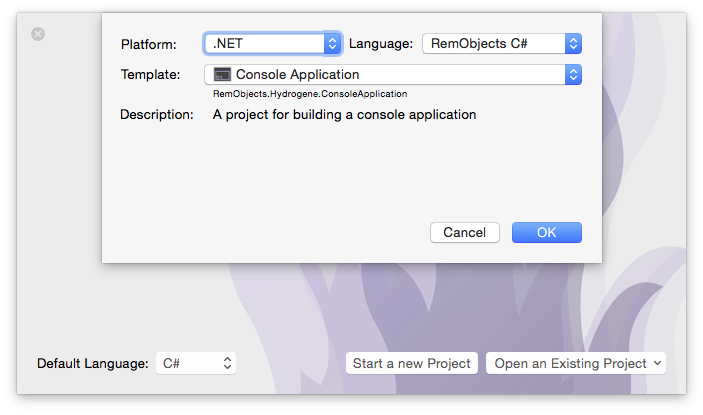
You will see that your preferred language is already pre-selected at the top right – although you can of course always choose a different language just for this one project.
On the top left you will select the platform for your new application. Since you're going to build a .NET app, select that to filter the third list down to show all .NET templates only. Drop down the big popup button in the middle and choose the "Console Application" project template, then click "OK".
Note: For this tutorial, we'll be building a command line app that can be easily run on Mac (and Windows and Linux). While Elements has full support for building GUI applications using WinForms (Oxygene only), WPF and WinRT, as well as for Windows Phone, the designers for these are not available in Fire. We recommend to use Visual Studio to build Windows GUI apps, and we have matching tutorials here.
Next, you will select where to save the new project you are creating:
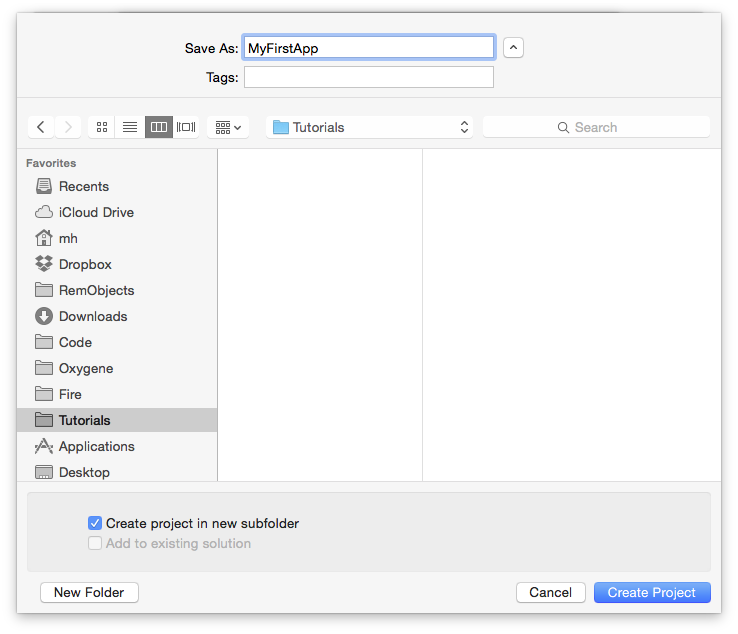
This is pretty much a standard Mac OS X Save Dialog; you can ignore the two extra options at the bottom for now and just pick a location for your project, give it a name, and click "Create Project".
You might be interested to know that you can set the default location for new projects in Preferences. Setting that to the base folder where you keep all your work, for example, saves you from having to find the right folder each time you start a new project.
Once the project is created, Fire opens its main window, showing you a view of your project:
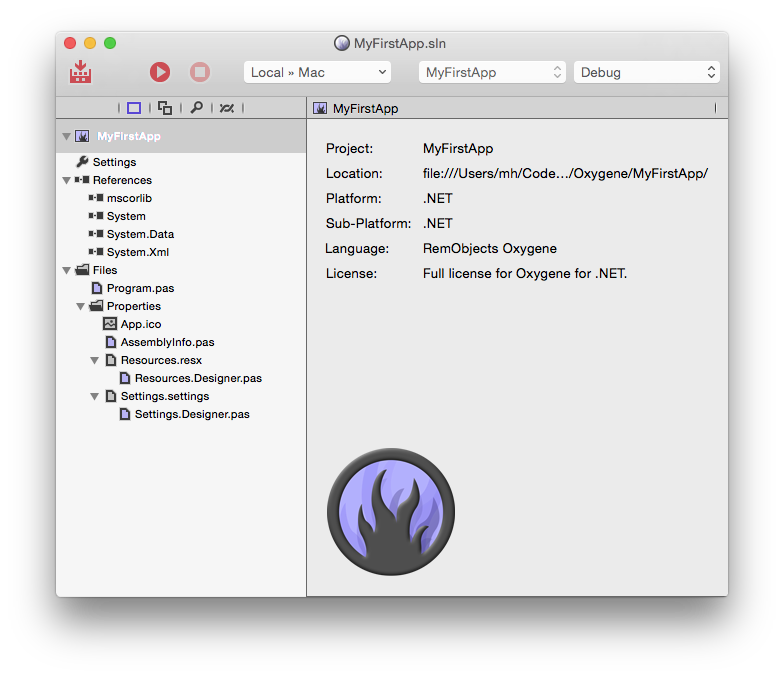
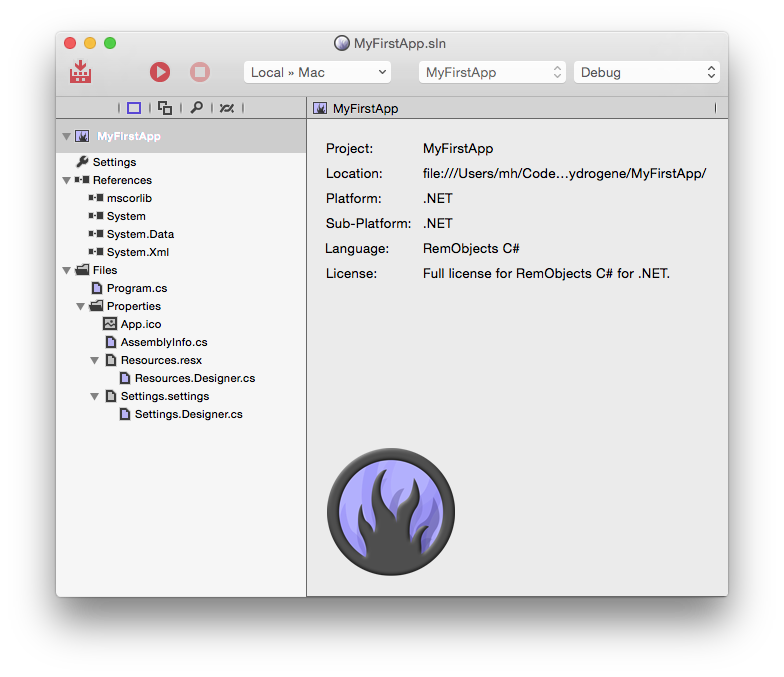
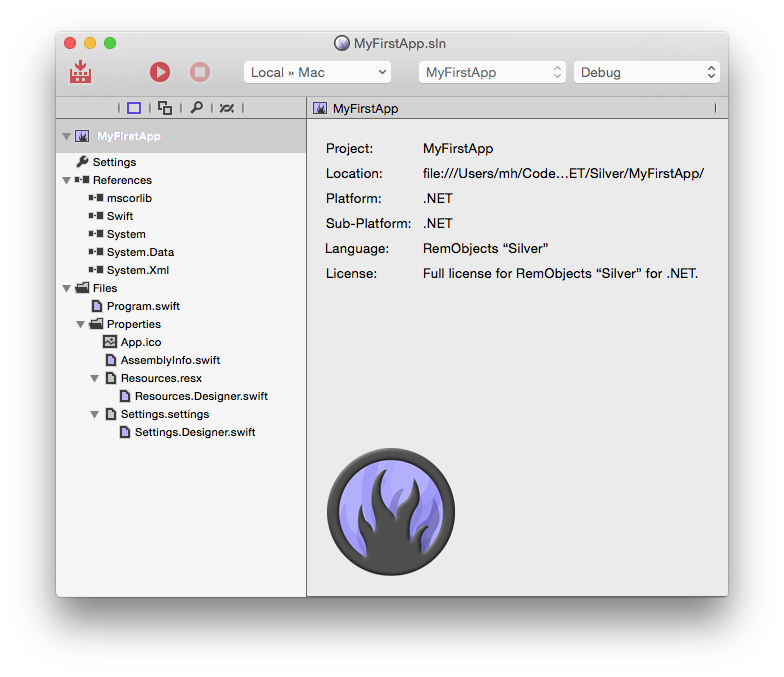
Let's have a look around this window, as there are a lot of things to see, and this windows is the main view of Fire where you will do most of your work.
The Fire Main Window
At the top, you will see the toolbar, and at the very top of that you see the name MyFirstApp.sln
. Now, MyFirstApp
is the name you gave your project, but what does .sln
mean? Elements works with projects inside of a Solution. You can think of a Solution as a container for one or more related projects. Fire will always open a solution, not a project – even if that solution might only contain a single project, like in this case.
In the toolbar itself are buttons to build and run the project, as well as a few popup buttons that let you select various things. We'll get to those later.
The left side of the Fire window is made up by what we call the Navigation Pane. This pane has various tabs at the top that allow you to quickly find your way around your project in various views. For now, we'll focus on the first view, which is active in the screenshot above, and is called the Project Tree.
You can hide and show the Navigation Pane by pressing ⌘0 at any time to let the main view (wich we'll look at next) fill the whole window. You can also bring up the Project Tree at any time by pressing ⌘1 (whether the Navigation Pane is hidden or showing a different tab).
The Project Tree
The Project Tree shows you a full view of everything in your project (or projects). Each project starts with the project node itself, which is larger, and selected in the screenshot above, as indicated by its blue background. Because it is selected, the main view on the right shows the project summary. As you select different nodes in the Project Tree the main view adjusts accordingly.
Each project has three top level nodes.
-
Settings gives you access to all the project settings and options for the project. Here you can control how the project is built and run, what exact compiler options are used, etc. The project settings are covered in great detail here.
-
References lists all the external frameworks and libraries your project uses. As you can see in the screenshot, the project already references all the most crucial libraries by default (we'll have a look at these later), and you can always add more by right-clicking the References node and choosing "Add Reference" from the context menu. You can also drag references in directly from the Finder, as well as, of course, remove unnecessary references. Please refer to the References topic for more in-depth coverage.
-
Files, finally, has the meat of your application. This is where all the files that make up your app are listed, including source files, images and other resources.
The Main View
Lastly, the main view fills the rest of the window (and if you hide the Navigation Pane, all of the window), and this is where you get your work done. With the project node selected, this view is a bit uninspiring, but when you select a source file, it will show you the code editor in that file, and it will show specific views for each file type.
When you hide the Navigation Pane, you can still navigate between the different files in your project via the Jump Bar at the top of the main view. Click on the "MyFirstApp" project name, and you can jump through the full folder and file hierarchy of your project, and more.
Your First .NET Project
Let's have a look at what's in the project that was generated for you from the template. This is already a fully working app that you could build and launch now – it wouldn't do much, but all the basic code and infrastructure is there.
There are two parts that are interesting. First, there's the Program
source file. This file serves as your program entry point, and has the so-called main()
function where execution starts. Secondly, there's a Properties
folder with a whole bunch of files that provide additional details and configuration for your project. The files in here will be common for all .NET projects.
The Properties Folder
If you want to start coding right away, you can, for now, pretty much ignore the files that are in here and skip to the next section. But in the interest of making you familiar with how .NET projects work, let's have a brief look.
Essentially, there are four main files in this folder, two of which have a nested .Designer
code file associated with them.
-
App.ico
, simple enough, is the icon for your executable, in standard Windows Icon format. Different than Mac apps, in .NET even command line executables can have an icon (although you will never see it anywhere, except on Windows), as the icon gets embedded directly into the executable file. The name of this file is not magic or hardcoded – it is referenced from the Project Settings. -
AssemblyInfo.*
is a code file that contains some standard Attributes that define metadata for your executable. These attributes can, for example, set a description and copyright message, or give the.exe
a Strong Name via code signing. There's typically no code in this file that will run (although nothing keeps you from adding some, or from moving the attributes out to a different code file). -
Resources.resx
is a .NET resource file in XML format that can be used to add resources such as images or strings to the project in a way that can make them easily localizable, and easily accessible from code. The file has a nestedResources.Designer.*
code file that will get updated automatically as the.resx
changes, and provides direct access to the resources from code, via a class calledMyFirstApp.Properties.Resources
. -
Settings.settings
is another XML file, this one allowing you to define configurable settings for your project. Imagine your app needed the URL of a server to talk to. You cold define a setting for that URL here, along with a default, and read that from code. Users of your app could later override the URL by providing a.config
file next to your executable where they provide a different value. Like the.resx
, this file has a nestedSettings.Designer.*
code file that will get updated automatically as the.settings
changes, and provides direct access to the resources from code, via a class calledMyFirstApp.Properties.Settings
.
For the purpose of this tutorial, you can ignore all of these files and move on to the code in Program
.
Program and the main()
Entry Point
The Program
source file is where the execution of your app starts and where (currently all) of the app's code lives. As mentioned before, the entry point is sometimes also referred to as the main()
function. Let's have a look.
In Oxygene and C#, the entry point is provided quite literally by a static method called Main()
that matches a well-defined signature: It takes an Array of Strings as parameter and optionally returns an Int32.
The string array will contain any parameters passed to the program from the command line, or will be empty if the program is called without (note that on .NET, the parameters do not include the executable name as first parameter, unlike native Mac console apps).
In Swift, the entry point looks a bit different. Any one single file in a Swift project can just contain code that's not contained in any class, and that code will be treated as the entry point. So Program.swift
defines no explicit class or main()
method, and instead just a line of code. If needed, the command line parameters can be accessed via the global C_ARGV
variable, which is a [String]
array, and their count can be accessed via C_ARGC
.
class method Program.Main(args: array of String): Int32;
begin
writeLn('The magic happens here.');
end;
public static Int32 Main(string[] args)
{
Console.WriteLine("The magic happens here.");
return 0;
}
println("The magic happens here.")
As you can see, the default implementation of the entry point does one thing: print out The magic happens here.
to the console.
The templates for the five languages use a different method for this, but this is purely a matter of taste or preference:
The C# snippet uses Console.WriteLine
, which is the official .NET API for talking to the console. The static Console
class has many functions that allow your code to interact with the terminal, including Console.ReadLine
to read input, as well.
The Oxygene template uses writeLn()
, which is a System Function, and the "classic" way for Pascal to print to the console. writeLn()
(and its counterpart write()
) are available to all languages and on all platforms, so using writeLn()
instead of Console.WriteLine
is a good way to write code that can cross-compile to Java or a native mac console app, too.
The Swift code uses println()
which is a standard Swift API, defined in the Swift Base Library. Like writeLn()
it works on all platforms, but is only available in Swift (or any project that uses the Swift Base Library).
The templates here differ merely to reflect the default that developers of each language would expect.
Without any further ado, you should now be ready to run this (very simple) first command line app.
Running Your App
As you might expect, .NET apps will require Mono to be installed in order to run on Mac OS X (or Linux). While Fire comes with its own copy of Mono for internal use, to run and debug apps on Mono, you will need to install the full Mono runtime on the system globally. The Mono runtime is available from mono-project.com, and we cover this in more detail in the Setup section, here.
With Mono installed, you can just hit the "Play" button in the toolbar, or press ⌘R.
Fire will now build your project and launch it. Since it is a console application, no UI will show up. Instead, Fire will capture its output live and show it in the Debug Console at the bottom of the window, which should automatically open up as soon as there is output, but can also be brought up manually by pressing ⌥⌘:
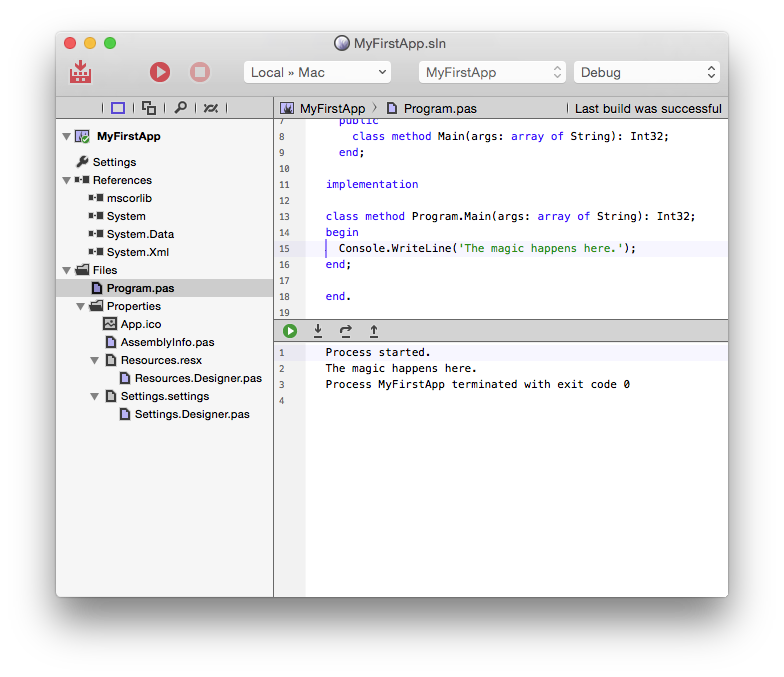
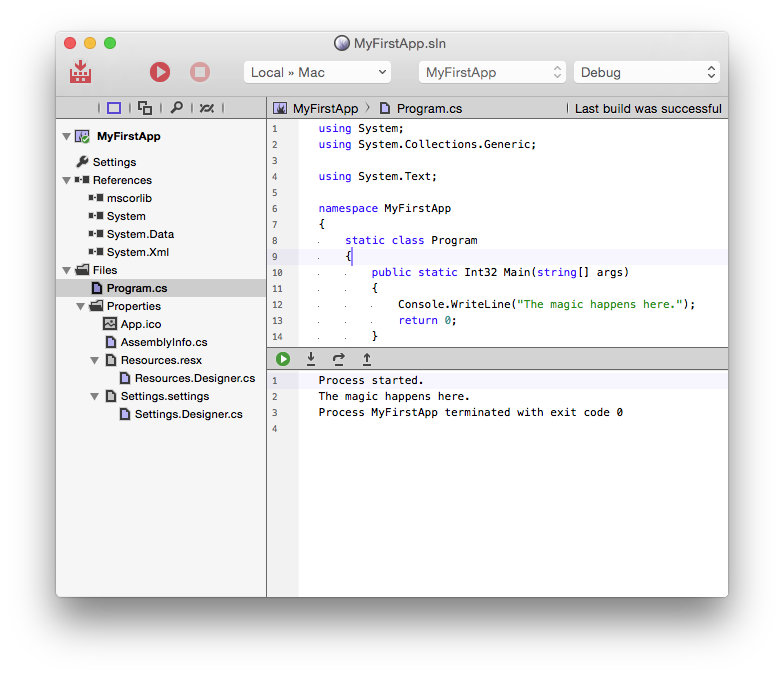
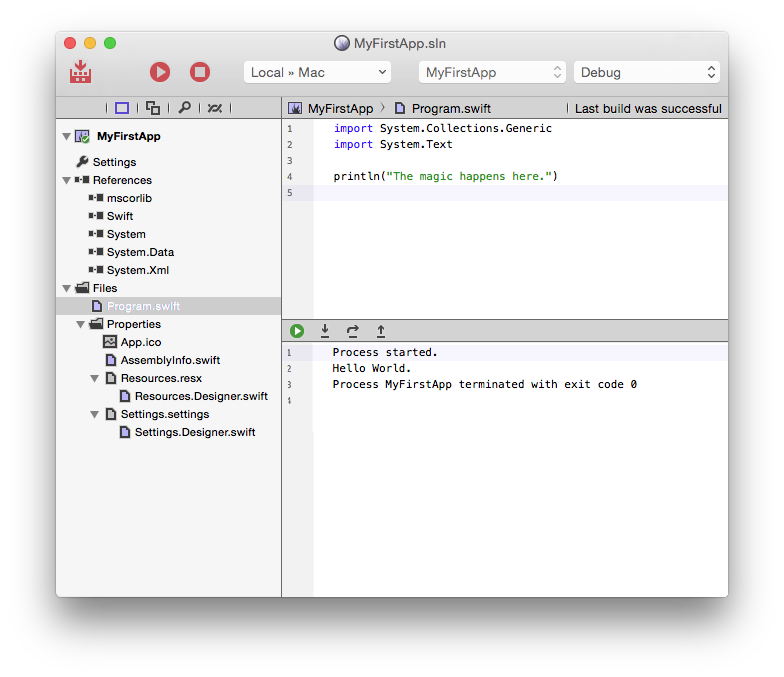
If you prefer to run your app in the Mac OS X Terminal, you can do so by pressing ⇧ ⌘R or use the "Project|Run w/o Debugging" menu command:
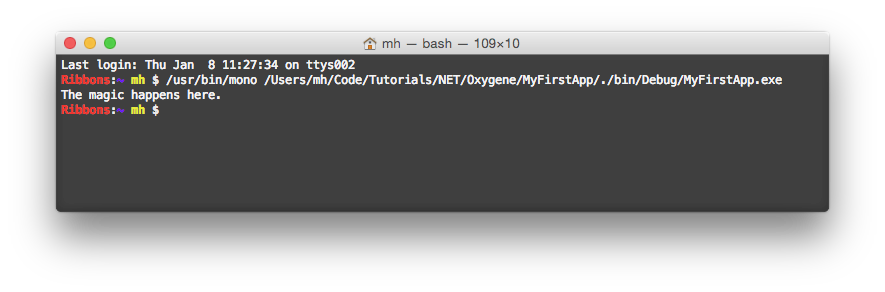
There are a couple of helpful hints for working with command line apps.
If you right-click the project node in the Project Tree, you will find an "Open Output Folder in Terminal" option in the context menu. As the name indicates, this will give you a new Terminal.app window that's already cd
ed to the right folder containing your executable. This way you can easily run it (for example with custom parameters) manually, or do other work with the exe.
For .NET apps, remember to prefix the app with the mono
command to run it, e.g.:
mono MyFirstApp.exe SomeParameter
Finally, you can configure command line parameters in Project Settings that Fire will use when running your app (both in the debugger and outside).
Running .NET Console Apps Cross-Platform
You will be able to take this same executable you built on the Mac and also run it Linux systems (via Mono) and on Windows (which has .NET support build in since Windows XP), as well. This is great for writing cross-platform command line tools or servers.
Of course Elements also lets you write Mac-native and Java Console apps as well, which we have covered in separate tutorials here.